Rust actix-web with maud
How to use maud in actix web as a lightweight template engine.
this is a working html page handler:
use actix_web::{get, web, Result};
use maud::{html, Markup};
#[get("/")]
async fn index() -> Result<Markup> {
// Fetch some data - this might fail, therefore using `Result` as function return value
let page_title = await fetch_title()
.map_err(|_| actix_web::error::ErrorInternalServerError("could not fetch categories"))?;
// render response
Ok(html! {
(maud::DOCTYPE)
html {
head {
title { (page_title) }
}
body {
main class="container flex-shrink-0 py-5" {
h1 { (page_title) }
}
}
}
})
}
and this is how you mount it as usual:
#[actix_web::main]
async fn main() -> std::io::Result<()> {
HttpServer::new(|| App::new().service(index))
.bind(("127.0.0.1", 8080))?
.run()
.await
}
Linked Technologies
What it's made of
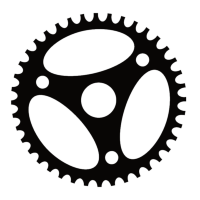
Actix-Web
Actix Web is a powerful, pragmatic, and extremely fast web framework for Rust. It runs on top of tokio and is probably the most mature rust web framework, with lots of available crates.
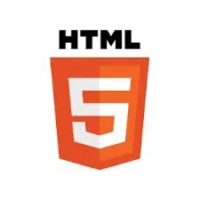
HTML
The backbone of the web. Master it to craft structured, accessible content for any online project, from simple sites to complex applications!
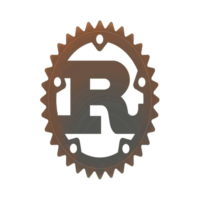
Rust
A language empowering everyone to build reliable and efficient software. Futuristic swiss army knife and probably the most powerful mainstream technology today
Linked Categories
Where it's useful
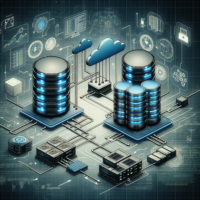
Backend Development
Uncover the power behind the scenes of web services and applications, focusing on creating robust, scalable backend systems that support frontend experiences.
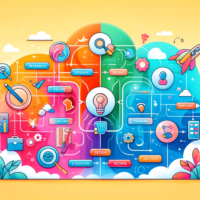
Frontend Development
Explore the latest tools and techniques for crafting responsive designs, enhancing user interfaces, and optimizing web performance.