Meteor React Login Form
The minimal working example to sign in a user with meteor accounts-password and React.js. Written in Typescript.
The bare minimum to get started with meteor accounts-password in typescript/react (TSX).
This is the sign in form to login an already registered user with a password. See the sign up form for the complementary registration snippet.
import { Meteor } from 'meteor/meteor';
import React, { useState } from 'react';
export const LoginForm = () => {
const [email, setEmail] = useState('');
const [password, setPassword] = useState('');
const submit = (e: React.FormEvent) => {
e.preventDefault();
Meteor.loginWithPassword({ email }, password);
};
return (
<form onSubmit={submit} className="login-form">
<label htmlFor="email">Email</label>
<input
type="text"
placeholder="Email"
name="email"
required
onChange={e => setEmail(e.target.value)}
/>
<label htmlFor="password">Password</label>
<input
type="password"
placeholder="Password"
name="password"
required
onChange={e => setPassword(e.target.value)}
/>
<button type="submit">Login</button>
</form>
);
};
If you also use Bootstrap and probably also react-bootstrap, this is a visually optimized version:
import { Meteor } from 'meteor/meteor';
import React, { useState } from 'react';
import { Form, Button } from 'react-bootstrap';
export const LoginForm = () => {
const [email, setEmail] = useState('');
const [password, setPassword] = useState('');
const submit = (e: React.FormEvent) => {
e.preventDefault();
Meteor.loginWithPassword({ email }, password);
};
return (
<Form onSubmit={submit}>
<Form.Group className="mb-3" controlId="formBasicEmail">
<Form.Label>Email address</Form.Label>
<Form.Control type="email" placeholder="Enter email" required onChange={e => setEmail(e.target.value)} />
</Form.Group>
<Form.Group className="mb-3" controlId="formBasicPassword">
<Form.Label>Password</Form.Label>
<Form.Control type="password" placeholder="Password" required onChange={e => setPassword(e.target.value)} />
</Form.Group>
<Button variant="primary" type="submit">
Login
</Button>
</Form>
);
};
Linked Technologies
What it's made of
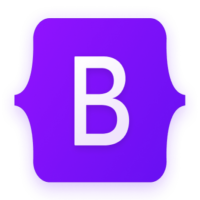
Bootstrap
One of the best and popular frontend component libraries in existence. While not the "hip" thing anymore, it gets the job done quickly and there are loads of learning resources and plugins.
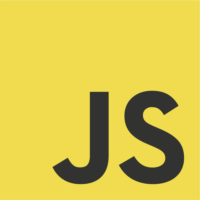
Javascript
Power up your web projects! Essential for dynamic interactions and seamless user experiences. A must-have for every developer's toolkit.
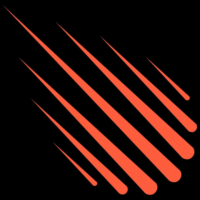
Meteor
Streamline app development with this full-stack platform. Rapid prototyping, real-time updates, and integrated tools make it ideal for startups.
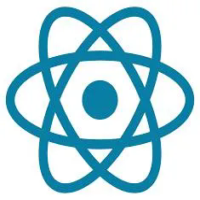
React
React.js: Build dynamic and responsive UIs with ease. Perfect for indie hackers looking to create scalable, efficient web applications!
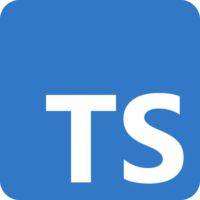
Typescript
Supercharge your JavaScript with strong typing. Ensure more reliable code and robust applications with this scalable, developer-friendly language.
Linked Categories
Where it's useful
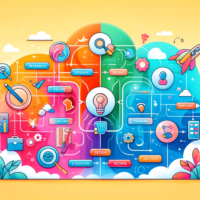
Frontend Development
Explore the latest tools and techniques for crafting responsive designs, enhancing user interfaces, and optimizing web performance.