Rust Chrono Relative Time Ago
A quick and dirty solution to get that "X days ago" String out of a chrono NaiveDateTime without any dependencies. Very easy to customize on-demand in your project!
use chrono::{NaiveDateTime, Utc};
pub fn time_ago(date: NaiveDateTime) -> String {
let now = Utc::now().naive_utc();
let duration = now.signed_duration_since(date);
match duration.num_days() {
0 => "today".to_string(),
1 => "yesterday".to_string(),
2..=6 => format!("{} days ago", duration.num_days()),
7..=13 => "1 week ago".to_string(),
14..=20 => "2 weeks ago".to_string(),
21..=27 => "3 weeks ago".to_string(),
28..=30 => "1 month ago".to_string(),
31..=59 => format!("{} months ago", duration.num_days() / 30),
60..=364 => format!("{} months ago", duration.num_days() / 30),
365..=729 => "1 year ago".to_string(),
_ => format!("{} years ago", duration.num_days() / 365),
}
}
Linked Technologies
What it's made of
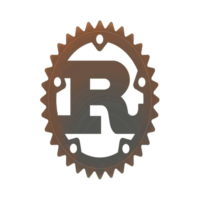
Rust
A language empowering everyone to build reliable and efficient software. Futuristic swiss army knife and probably the most powerful mainstream technology today
Linked Categories
Where it's useful
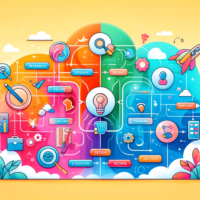
Frontend Development
Explore the latest tools and techniques for crafting responsive designs, enhancing user interfaces, and optimizing web performance.