React Pagination Hook
Drop-in typescript code to handle pagination properly with react hooks and URL query parameters
This short hook leverages several best practices:
- use an URL query parameter to persist the current state
- better UX when reloading the website
- better SEO since all pages are crawlable
- leverage
history.replace()
to preserve clean browser history - API surface is the same as
useState()
- graceful fallback to
1
if there is no page parameter set currently
import { useHistory, useLocation } from "react-router-dom";
export const usePagination = (): [number, (page: number) => void] => {
const { pathname, search } = useLocation();
const params = new URLSearchParams(search);
const page = parseInt(params.get('page') || "1");
const history = useHistory();
const setPage = (newPage = 1) => {
params.set('page', newPage.toString())
history.replace({ pathname, search: params.toString() });
}
return [page, setPage]
}
Linked Technologies
What it's made of
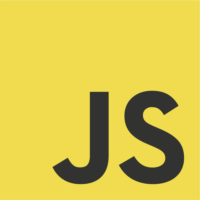
Javascript
Power up your web projects! Essential for dynamic interactions and seamless user experiences. A must-have for every developer's toolkit.
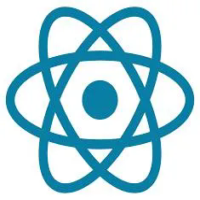
React
React.js: Build dynamic and responsive UIs with ease. Perfect for indie hackers looking to create scalable, efficient web applications!
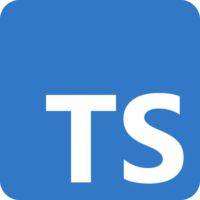
Typescript
Supercharge your JavaScript with strong typing. Ensure more reliable code and robust applications with this scalable, developer-friendly language.
Linked Categories
Where it's useful
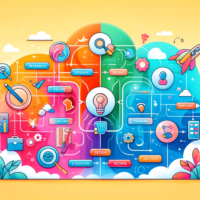
Frontend Development
Explore the latest tools and techniques for crafting responsive designs, enhancing user interfaces, and optimizing web performance.